Data Structure & Algorithm
Categories: Job Ready Course
About Course
Data Structures and Algorithms (DSA) is a foundational area of computer science that focuses on organizing data efficiently and solving problems through step-by-step procedures. Data structures like arrays, linked lists, stacks, and trees are used to store and manage data, while algorithms such as sorting, searching, and recursion provide techniques to process that data. Mastery of DSA is essential for efficient problem-solving and is widely used in software development and technical interviews.
What Will You Learn?
- Fundamental data structures like arrays, linked lists, stacks, and queues.
- Advanced data structures such as trees, graphs, heaps, and hash tables.
- Searching and sorting algorithms (e.g., binary search, quicksort, mergesort).
- Concepts of time and space complexity (Big O notation).
- Recursion and backtracking techniques.
- Greedy algorithms and dynamic programming.
- Graph algorithms including depth-first search (DFS) and breadth-first search (BFS).
- Practical problem-solving using data structures and algorithms for real-world applications.
Course Content
Introduction
-
Introduction to DSA
00:00 -
Why Learn DSA?
00:00 -
Prerequisites
00:00 -
Python Lists
00:00
Sorting Algorithms
-
Sorting
00:00 -
Bubble Sort
00:00 -
Selection Sort
00:00 -
Insertion Sort
00:00 -
Merge Sort
00:00 -
Quick Sort
00:00 -
Counting Sort
00:00
Search Algorithms
-
Search Algorithms
00:00 -
Linear Search
00:00 -
Binary Search
00:00
Linked List
-
Linked List
00:00 -
Linked List Operations
00:00 -
Examples: Linked List
00:00
Circular Linked List
-
Introduction to Circular Linked List
00:00 -
Circular Linked List Operations
00:00 -
Examples: Circular Linked List
00:00
Doubly Linked List
-
Introduction to Doubly Linked List
00:00 -
Doubly Linked List Operations
00:00 -
Examples: Doubly Linked List
00:00
Stacks and Queue
-
Stack
00:00 -
Queue
00:00 -
Drawbacks of Linear Queues
00:00 -
Circular Queue
00:00 -
Double Ended Queue (Deque)
00:00
Hashing
-
Introduction to Hashing
00:00 -
Hashing Techniques
00:00 -
Hash Collision
00:00 -
Hash Collision Resolution
00:00 -
Hash Functions8.6Additional Topics
00:00
String Matching Algorithms
-
Introduction
00:00 -
Brute Force Method
00:00 -
Rabin-Karp String Matching Algorithm
00:00 -
Knuth-Morris-Pratt (KMP) Algorithm
00:00
Tree Data Structure
-
Non-linear Data Structure
00:00 -
Tree Data Structure
00:00 -
Implementation of Trees
00:00 -
Tree Traversal
00:00
Binary Tree
-
Introduction to Binary Trees
00:00 -
Properties of Binary Trees
00:00 -
Implementation of Binary Trees
00:00 -
Traversal of Binary Tree
00:00 -
Types of Binary Trees
00:00 -
Example: Huffman Coding
00:00 -
Example: Binary Search Tree
00:00
Heaps
-
Array Representation of Binary Tree
00:00 -
Introduction to Heaps
00:00 -
Heap Operations
00:00 -
Heapify
00:00 -
Heap Sort
00:00 -
Heap as a Priority Queue
00:00
Graph Data Structure
-
Graph Data Structure
00:00 -
Graph Terminologies
00:00 -
Adjacency Matrix
00:00 -
Adjacency List
00:00 -
Graph Traversal With DFS Algorithm
00:00 -
BFS Algorithm
00:00
Relationships in a Graph
-
Graph Connectivity
00:00 -
Touring a Graph
00:00 -
Graph Comparisons and Special Properties
00:00 -
Types of Graph
00:00
Graph Based Algorithms
-
Graph Based Algorithms
00:00 -
Topological Sorting
00:00 -
Dijkstra’s Algorithm
00:00 -
Bellman Ford’s Algorithm
00:00 -
Ford Fulkerson Algorithm
00:00 -
Spanning Trees
00:00 -
Kruskal’s Algorithm
00:00 -
Prim’s Algorithm
00:00
Greedy Algorithms
-
Introduction to Greedy Algorithms
00:00 -
The Classroom Scheduling Problem
00:00 -
Coin Change Problem
00:00 -
The Fractional Knapsack Problem
00:00 -
0-1 Knapsack Problem
00:00
Sorting (II)
-
Additional Sorting Techniques
00:00 -
Bucket Sort
00:00 -
Radix Sort
00:00 -
Shell Sort
00:00
Balanced Trees
-
Introduction to Balanced Trees
00:00 -
AVL Trees
00:00 -
Red-Black Trees
00:00
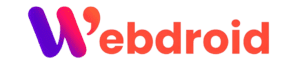
Elevate Your Learning Journey with Cutting-Edge Education Technology.
Company
- Contact
- About
- Tech Blog
- Self-Paced Courses
- Events
Our Programs
- Data Analytics
- Digital Marketing
- Web Development
- Cyber Security
- App Development
- Design
Digital Marketing Courses
Digital Marketing Course | SEO Course | Social Media Marketing Course | Content Writing Course | YouTube Course | Instagram Marketing Course | Google Ads Course | Copywriting Course | Performance Marketing Course
Web Development Courses
Full Stack Developer Course | WordPress Course | MERN Stack Course | Laravel Course | Web Development Course | HTML Course | ReactJS Course | Javascript Course | PHP Course | NodeJS Course | Front-End Development Course | Web Designing Course | CSS Course
More Professional Courses
Cyber Security Course | Tableau Course | Android App Development Course | Mobile App Development Course | Power BI Course
Free Courses
Semrush Course | Google Tag Manager Course | Blogging Course | Photoshop Course | Video Editing Course | AngularJS Course | Shopify Course | Django Course | Email Marketing Course | Affiliate Marketing Course
Interview Questions
HTML Interview Questions | CSS Interview Questions | PHP Interview Questions | JavaScript Interview Questions | Flutter Interview Questions | Data Structure Interview Questions | Java Interview Questions | MySQL Interview Questions | Python Interview Questions | DBMS Interview Questions | Power BI Interview Questions | Angular Interview Questions | ReactJS Interview Questions | C Interview Questions | Django Interview Questions | Email Marketing Interview Questions | Content Writing Interview Questions | NodeJS Interview Questions | SEO Interview Questions | OOPS Interview Questions | SQL Interview Questions | Digital Marketing Interview Questions
Popular Career Resources
Professional Courses After 12th | Courses After Graduation | How to Become SEO Freelancer? | High-Income Skills | Digital Marketing Books | Become Google Ads Expert | Build a Career in Digital Marketing | SEO Career Path | Make Money Online | Become Data Analyst | Become a Flutter Developer | Best Programming Languages to Learn | Become Ethical Hacker | Python Developer Salary | Full Stack Developer Salary | Data Analyst Salary | Free Digital Marketing Projects