Competitive Programming
Categories: Job Ready Course
About Course
Competitive programming is a coding discipline where participants solve algorithmic and mathematical problems within a limited time frame. It focuses on efficient problem-solving using data structures, algorithms, and optimization techniques. Competitions often take place on online platforms, where coders are ranked based on the speed and accuracy of their solutions. This practice enhances logical thinking, coding skills, and the ability to perform under pressure.
What Will You Learn?
- Problem-solving techniques using algorithms and data structures.
- How to optimize code for time and space efficiency.
- Implementation of algorithms like sorting, searching, and graph theory.
- Advanced topics such as dynamic programming, greedy algorithms, and backtracking.
- Debugging and testing strategies to ensure correctness.
- Efficient coding practices and syntax in programming languages like C++, Java, or Python.
- Techniques to approach complex problems under time constraints.
- Strategies for participating in coding competitions and challenges.
Course Content
Introduction to Competitive Programming
-
Introduction to the Course
00:00 -
What is Competitive Programming
00:00 -
Competitive Programming Competitions
00:00 -
Websites for Competitive Programming
00:00 -
Terminologies in Competitive Programming
00:00 -
Choosing a Programming Language
00:00 -
Taking Input & Output in Competitive Programming Contests
00:00 -
Cout vs Printf
00:00 -
Endl vs Back n
00:00 -
Fast Cin Cout Methods _ Conclusion
00:00 -
Time Complexity in Competitive Programming
00:00 -
Calculating Time Complexity of Code
00:00 -
Importance of English in Competitive Programming
00:00
Standard Template Library (STL)
-
Introduction to STL in C++
00:00 -
Standard Array
00:00 -
Standard Vector
00:00 -
Standard Set
00:00 -
Standard Multiset
00:00 -
Standard Map
00:00 -
Standard Multimap
00:00 -
Standard Pair
00:00 -
Standard Forward List
00:00 -
Standard List
00:00 -
Standard Unordered Set
00:00 -
Standard Unordered Multiset
00:00 -
Unordered Map and Unordered Multimap
00:00 -
Standard Stack
00:00 -
Standard Queue
00:00 -
Standard Priority Queue
00:00 -
Standard Deque
00:00
Bit Manipulation and Modulo Arithmetic
-
Importance of Bit Manipulation Techniques
00:00 -
Basic Binary Operations
00:00 -
Bit Operations in C++
00:00 -
Check ith bit is SET or NOT SET
00:00 -
Toggle ith bit
00:00 -
Check odd or even using bits
00:00 -
Check if Number is Power of 2
00:00 -
Need for Modulo 10^9 + 7
00:00 -
Modulo Arithmetic
00:00 -
[A3.1] Problem XOR ORED
00:00 -
[A3.2] Solution – XOR-ORED
00:00 -
[A3.3] Solution with Code – XOR-ORED
00:00
Graphs
-
Introduction to Graphs
00:00 -
Weights in Graphs
00:00 -
Why Study Graphs
00:00 -
Edge List
00:00 -
Adjacenty Matrix
00:00 -
Adjacenty List
00:00 -
DFS Traversal
00:00 -
Implementing DFS Traversal
00:00 -
BFS Traversal
00:00 -
BFS Traversal
00:00 -
Minimum Spanning Tree
00:00 -
Concept of Kruskal_s Algorithm
00:00 -
Concept of Prim_s Algorithm
00:00 -
Concept of Dijkstra Algorithm
00:00 -
[A4.1] Problem – CHFPLN
00:00 -
[A4.2] Solution – CHFPLN
00:00 -
[A4.2] Solution – CHFPLN
00:00
Segment Tree
-
Sum of Updated Range Problem
00:00 -
Solving Sum of Range Problem with Segment Trees
00:00 -
Segment Trees – Update Queries
00:00 -
Segment Trees – Calculate Sum
00:00 -
Implementing Segment Tree – Part 1
00:00 -
Implementing Segment Tree – Part 2
00:00 -
Problem – Range Minimum Query
00:00 -
Implementing Range Minimum Query
00:00 -
Implementing Segment Tree – Tricks
00:00 -
Lazy Propagation in Segment Trees – Part 1
00:00 -
Lazy Propagation in Segment Trees – Part 2
00:00 -
Implementing Lazy Propagation in Segment Trees – Part 1
00:00 -
Implementing Lazy Propagation in Segment Trees – Part 2
00:00 -
Problem – Xenia and Bit Operations
00:00 -
Solution – Xenia and Bit Operations
00:00 -
Solution with Code – Xenia and Bit Operations
00:00 -
Problem – Circular RMQ
00:00 -
Solution with Code – Circular RMQ – Part 1
00:00 -
Solution with Code – Circular RMQ – Part 2
00:00
Backtracking
-
Introduction to Backtracking
00:00 -
N Queens Problem Explained
00:00 -
N Queens Solved
00:00 -
Knights Tour Problem Explained
00:00 -
Knight’s Tour Solved
00:00 -
Rat in Maze Problem Explained
00:00 -
Rat in Maze Solved
00:00 -
Problem Richie Rich
00:00 -
Solving Richie Rich
00:00 -
Problem False Number
00:00 -
Solving False Number
00:00
Greedy Algorithms
-
Algorithms
00:00 -
Introduction to Greedy Algorithms
00:00 -
Understanding Greedy with Fractional Knapsack Problem
00:00 -
Solving Fractional Knapsack Problem
00:00 -
Tasks and Deadlines Problem
00:00 -
Solving Tasks and Deadlines Problem
00:00 -
Optimal File Merging Problem
00:00 -
Solving Optimal File Merge Patterns Problem
00:00 -
Huffman Coding
00:00 -
[A7.1] Problem – Dragons
00:00 -
[A7.2] Solution with Code – Dragons
00:00 -
[A7.3] Problem – Little Elephant and Bits
00:00 -
[A7.4] Solution with Code – Little Elephant and Bits
00:00
Dynamic Programming
-
Introduction to Dynamic Programming
00:00 -
Top down vs Bottom Up
00:00 -
Fibbonacci Problem Top Down
00:00 -
Implementing Fibonnaci with DP
00:00 -
2D Grid Traversal Problem
00:00 -
Implementing 2D Grid Traversal Problem
00:00 -
Understanding Memoization
00:00 -
Fibbonacci with Tabulation
00:00 -
Implementing Fibbonacci with Tabulation
00:00 -
2D Grid Traversal Problem with Tabulation
00:00 -
Implementing 2D Grid Traversal Problem with Tabulation
00:00 -
Understanding Tabulation
00:00 -
[A8.1] Problem – Subset Sum Problem
00:00 -
[A8.2] Solving – Subset Sum Problem
00:00
Advanced Concepts
-
Binary Lifting (Kth Ancestor of a Tree Node)
00:00 -
LCA (Lowest Common Ancestor) Problem
00:00 -
Fenwick Trees – Introduction
00:00 -
Fenwick Trees – Sum of Range (Range Find Query)
00:00 -
Fenwick Trees – Update (Point Update Query)
00:00 -
Problem – Distance between nodes in Trees
00:00 -
Solution – Distance between nodes in Trees
00:00 -
Implementing Fenwick Trees
00:00 -
Implementing Binary Uplifting
00:00 -
Implementing LCA
00:00 -
Solution with Code – Distance between nodes in Trees
00:00
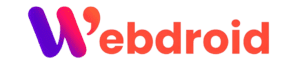
Elevate Your Learning Journey with Cutting-Edge Education Technology.
Digital Marketing Courses
Digital Marketing Course | SEO Course | Social Media Marketing Course | Content Writing Course | YouTube Course | Instagram Marketing Course | Google Ads Course | Copywriting Course | Performance Marketing Course
Web Development Courses
Full Stack Developer Course | WordPress Course | MERN Stack Course | Laravel Course | Web Development Course | HTML Course | ReactJS Course | Javascript Course | PHP Course | NodeJS Course | Front-End Development Course | Web Designing Course | CSS Course
More Professional Courses
Cyber Security Course | Tableau Course | Android App Development Course | Mobile App Development Course | Power BI Course
Free Courses
Semrush Course | Google Tag Manager Course | Blogging Course | Photoshop Course | Video Editing Course | AngularJS Course | Shopify Course | Django Course | Email Marketing Course | Affiliate Marketing Course
Interview Questions
HTML Interview Questions | CSS Interview Questions | PHP Interview Questions | JavaScript Interview Questions | Flutter Interview Questions | Data Structure Interview Questions | Java Interview Questions | MySQL Interview Questions | Python Interview Questions | DBMS Interview Questions | Power BI Interview Questions | Angular Interview Questions | ReactJS Interview Questions | C Interview Questions | Django Interview Questions | Email Marketing Interview Questions | Content Writing Interview Questions | NodeJS Interview Questions | SEO Interview Questions | OOPS Interview Questions | SQL Interview Questions | Digital Marketing Interview Questions
Popular Career Resources
Professional Courses After 12th | Courses After Graduation | How to Become SEO Freelancer? | High-Income Skills | Digital Marketing Books | Become Google Ads Expert | Build a Career in Digital Marketing | SEO Career Path | Make Money Online | Become Data Analyst | Become a Flutter Developer | Best Programming Languages to Learn | Become Ethical Hacker | Python Developer Salary | Full Stack Developer Salary | Data Analyst Salary | Free Digital Marketing Projects