Java Developer
About Course
A Java Developer specializes in creating applications with the Java programming language, known for its portability and performance. They develop robust software solutions that run smoothly across various platforms, including web applications and Android mobile apps. Using frameworks like Spring and Hibernate, they build scalable back-end systems that handle data processing and business logic efficiently. Their knowledge of object-oriented programming helps them write clean and maintainable code.
Additionally, Java Developers work with databases to integrate SQL or NoSQL solutions for effective data management. They often collaborate with front-end developers to create user-friendly interfaces, ensuring a cohesive user experience. Overall, their expertise in Java makes them vital for projects requiring reliable and high-performing software solutions.
What Will You Learn?
- Core Java programming concepts like OOP (Object-Oriented Programming), data types, and control structures.
- Advanced Java features like multithreading, collections, and exception handling.
- Java frameworks such as Spring and Hibernate for enterprise-level application development.
- Working with databases using JDBC or JPA for data persistence.
- Building RESTful APIs and web services in Java.
- Testing and debugging Java applications using tools like JUnit and Mockito.
- Version control and collaboration using Git and GitHub.
- Deployment and server management for Java applications with tools like Maven and Docker.
Course Content
Introduction
-
Java Program to Print an Integer (Entered by the User)
00:00 -
Java Program to Add Two Integers
00:00 -
Java Program to Multiply two Floating Point Numbers
00:00 -
Java Program to Find ASCII Value of a character
00:00 -
Java Program to Compute Quotient and Remainder
00:00 -
Java Program to Swap Two Numbers
00:00 -
Java Program to Check Whether a Number is Even or Odd
00:00 -
Java Program to Check Whether an Alphabet is Vowel or Consonant
00:00 -
Java Program to Find the Largest Among Three Numbers
00:00 -
Java Program to Find all Roots of a Quadratic Equation
00:00 -
Java Program to Find the Frequency of Character in a String
00:00 -
Java Program to Remove All Whitespaces from a String
00:00 -
Java Program to Round a Number to n Decimal Places
00:00 -
Java Program to Check if a String is Empty or Null
00:00
Type Conversion
-
Java Program to Convert Character to String and Vice-Versa
00:00 -
Java Program to convert char type variables to int
00:00 -
Java Program to convert int type variables to char
00:00 -
Java Program to convert long type variables into int
00:00 -
Java Program to convert int type variables to long
00:00 -
Java Program to convert boolean variables into string
00:00 -
Java Program to convert string type variables into boolean
00:00 -
Java Program to convert string type variables into int
00:00 -
Java Program to convert int type variables to String
00:00 -
Java Program to convert int type variables to double
00:00 -
Java Program to convert double type variables to int
00:00 -
Java Program to convert string variables to double
00:00 -
Java Program to convert double type variables to string
00:00 -
Java Program to convert primitive types to objects and vice versa
00:00
Decision Making and Loop
-
Java Program to Check Leap Year
00:00 -
Java Program to Check Whether a Number is Positive or Negative
00:00 -
Java Program to Check Whether a Character is Alphabet or Not
00:00 -
Draft LessonJava Program to Calculate the Sum of Natural Numbers
00:00 -
Java Program to Find Factorial of a Number
00:00 -
Java Program to Generate Multiplication Table
00:00 -
Java Program to Display Fibonacci Series
00:00 -
Java Program to Find GCD of two Numbers
00:00 -
Java Program to Find LCM of two Numbers
00:00 -
Java Program to Display Alphabets (A to Z) using loop
00:00 -
Java Program to Count Number of Digits in an Integer
00:00 -
Java Program to Reverse a Number
00:00 -
Java Program to Calculate the Power of a Number
00:00 -
Java Program to Check Palindrome
00:00 -
Java Program to Check Whether a Number is Prime or Not
00:00 -
Java Program to Display Prime Numbers Between Two Intervals
00:00 -
Java Program to Check Armstrong Number
00:00 -
Java Program to Display Armstrong Number Between Two Intervals
00:00 -
Java Program to Display Factors of a Number
00:00 -
Java Program to Make a Simple Calculator Using switch…case
00:00 -
Java Program to Count the Number of Vowels and Consonants in a Sentence
00:00 -
Java Program to Sort Elements in Lexicographical Order (Dictionary Order)
00:00 -
Java Code To Create Pyramid and Pattern
00:00
Functions
-
Java Program to Display Prime Numbers Between Intervals Using Function
00:00 -
Java Program to Display Armstrong Numbers Between Intervals Using Function
00:00 -
Java Program to Check Whether a Number can be Expressed as Sum of Two Prime Numbers
00:00 -
Java Program to Find the Sum of Natural Numbers using Recursion
00:00 -
Java Program to Find Factorial of a Number Using Recursion
00:00 -
Java Program to Find G.C.D Using Recursion
00:00 -
Java Program to Convert Binary Number to Decimal and vice-versa
00:00 -
Java Program to Convert Octal Number to Decimal and vice-versa
00:00 -
Java Program to Convert Binary Number to Octal and vice-versa
00:00 -
Java Program to Reverse a Sentence Using Recursion
00:00 -
Java Program to calculate the power using recursion
00:00 -
Java Program to Call One Constructor from another
00:00 -
Java Program to implement private constructors
00:00 -
Java Program to pass lambda expression as a method argument
00:00 -
Java Program to Pass the Result of One Method Call to Another Method
00:00 -
Java Program to Calculate the Execution Time of Methods
00:00
Array
-
Java Program to Calculate Average Using Arrays
00:00 -
Java Program to Find Largest Element of an Array
00:00 -
Java Program to Calculate Standard Deviation
00:00 -
Java Program to Add Two Matrix Using Multi-dimensional Arrays
00:00 -
Java Program to Multiply Two Matrix Using Multi-dimensional Arrays
00:00 -
Java Program to Multiply two Matrices by Passing Matrix to a Function
00:00 -
Java Program to Find Transpose of a Matrix
00:00 -
Java Program to Print an Array
00:00 -
Java Program to Concatenate Two Arrays
00:00 -
Java Program to Check if An Array Contains a Given Value
00:00
Object and Class
-
Java Program to Add Two Complex Numbers by Passing Class to a Function
00:00 -
Java Program to Calculate Difference Between Two Time Periods
00:00 -
Java Program to Determine the class of an object
00:00 -
Java Program to Create an enum class
00:00 -
Java Program to Print object of a class
00:00 -
Java Program to Create custom exception
00:00 -
Java Program to Create an Immutable Class
00:00
String
-
Java Program to Convert String to Date
00:00 -
Java Program to Convert a Stack Trace to a String
00:00 -
Java Program to Compare Strings
00:00 -
Java Program to Check if a String is Numeric
00:00 -
Java Program to Check if two strings are anagram
00:00 -
Java Program to Compute all the permutations of the string
00:00 -
Java Program to Create random strings
00:00 -
Java Program to Clear the StringBuffer
00:00 -
Java Program to Capitalize the first character of each word in a String
00:00 -
Java Program to Iterate through each characters of the string.
00:00 -
Java Program to Differentiate String == operator and equals() method
00:00 -
Java Program to Implement switch statement on strings
00:00 -
Java Program to Check if a string contains a substring
00:00 -
Java Program to Check if a string is a valid shuffle of two distinct strings
00:00
Collections
-
Java Program to Join Two Lists
00:00 -
Java Program to Convert a List to Array and Vice Versa
00:00 -
Java Program to Convert Map (HashMap) to List
00:00 -
Java Program to Convert Array to Set (HashSet) and Vice-Versa
00:00 -
Java Program to Sort a Map By Values
00:00 -
Java Program to Sort ArrayList of Custom Objects By Property
00:00 -
Java Program to Implement LinkedList
00:00 -
Java Program to Implement stack data structure
00:00 -
Java Program to Implement the queue data structure
00:00 -
Java Program to Get the middle element of LinkedList in a single iteration
00:00 -
Java Program to Convert the LinkedList into an Array and vice versa
00:00 -
Java Program to Convert the ArrayList into a string and vice versa
00:00 -
Java Program to Iterate over an ArrayList
00:00 -
Java Program to Iterate over a HashMap
00:00 -
Java Program to Iterate over a Set
00:00 -
Java Program to Merge two lists
00:00 -
Java Program to Update value of HashMap using key
00:00 -
Java Program to Remove duplicate elements from ArrayList
00:00 -
Java Program to Get key from HashMap using the value
00:00 -
Java Program to Detect loop in a LinkedList
00:00 -
Java Program to Calculate union of two sets
00:00 -
Java Program to Calculate the intersection of two sets
00:00 -
Java Program to Calculate the difference between two sets
00:00 -
Java Program to Check if a set is the subset of another set
00:00 -
Java Program to Sort map by keys
00:00 -
Java Program to Pass ArrayList as the function argument
00:00 -
Java Program to Iterate over ArrayList using Lambda Expression
00:00 -
Java Program to Implement Binary Tree Data Structure
00:00 -
Java Program to Perform the preorder tree traversal
00:00 -
Java Program to Perform the postorder tree traversal
00:00 -
Java Program to Perform the inorder tree traversal
00:00 -
Java Program to Count number of leaf nodes in a tree
00:00 -
Java Program to Implement the graph data structure
00:00 -
Java Program to Remove elements from the LinkedList.
00:00 -
Java Program to Add elements to a LinkedList
00:00 -
Java Program to Access elements from a LinkedList.
00:00
Algorithms
-
Java Program to Implement Bubble Sort algorithm
00:00 -
Java Program to Implement Quick Sort Algorithm
00:00 -
Java Program to Implement Merge Sort Algorithm
00:00 -
Java Program to Implement Binary Search Algorithm
00:00
Files
-
Java Program to Get Current Working Directory
00:00 -
Java Program to Create String from Contents of a File
00:00 -
Java Program to Append Text to an Existing File
00:00 -
Java Program to Convert File to byte array and Vice-Versa
00:00 -
Java Program to Create File and Write to the File
00:00 -
Java Program to Read the Content of a File Line by Line
00:00 -
Java Program to Delete File in Java
00:00 -
Java Program to Delete Empty and Non-empty Directory
00:00 -
Java Program to Get the File Extension
00:00 -
Java Program to Get the name of the file from the absolute path
00:00 -
Java Program to Get the relative path from two absolute paths
00:00 -
Java Program to Count number of lines present in the file
00:00
I/O Stream
-
Java Program to Convert InputStream to String
00:00 -
Java Program to Convert OutputStream to String
00:00 -
Java Program to Convert a String into the InputStream
00:00 -
Java Program to Convert the InputStream into Byte Array
00:00 -
Java Program to Load File as InputStream
00:00
Advanced
-
Java Program to Get Current Date/Time
00:00 -
Java Program to Convert Milliseconds to Minutes and Seconds
00:00 -
Java Program to Add Two Dates
00:00 -
Java Program to Convert Byte Array to Hexadecimal
00:00 -
Java Program to Lookup enum by String value
00:00 -
Java Program to Calculate simple interest and compound interest
00:00 -
Java Program to Implement multiple inheritance
00:00 -
Java Program to Determine the name and version of the operating system
00:00 -
Java Program to Check if two of three boolean variables are true
00:00 -
Java Program to Iterate over enum
00:00 -
Java Program to Check the birthday and print Happy Birthday message
00:00 -
Java Program to Access private members of a class
00:00
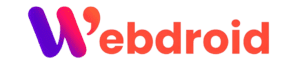
Elevate Your Learning Journey with Cutting-Edge Education Technology.